(2) Programming Fundamentals: Pseudocode
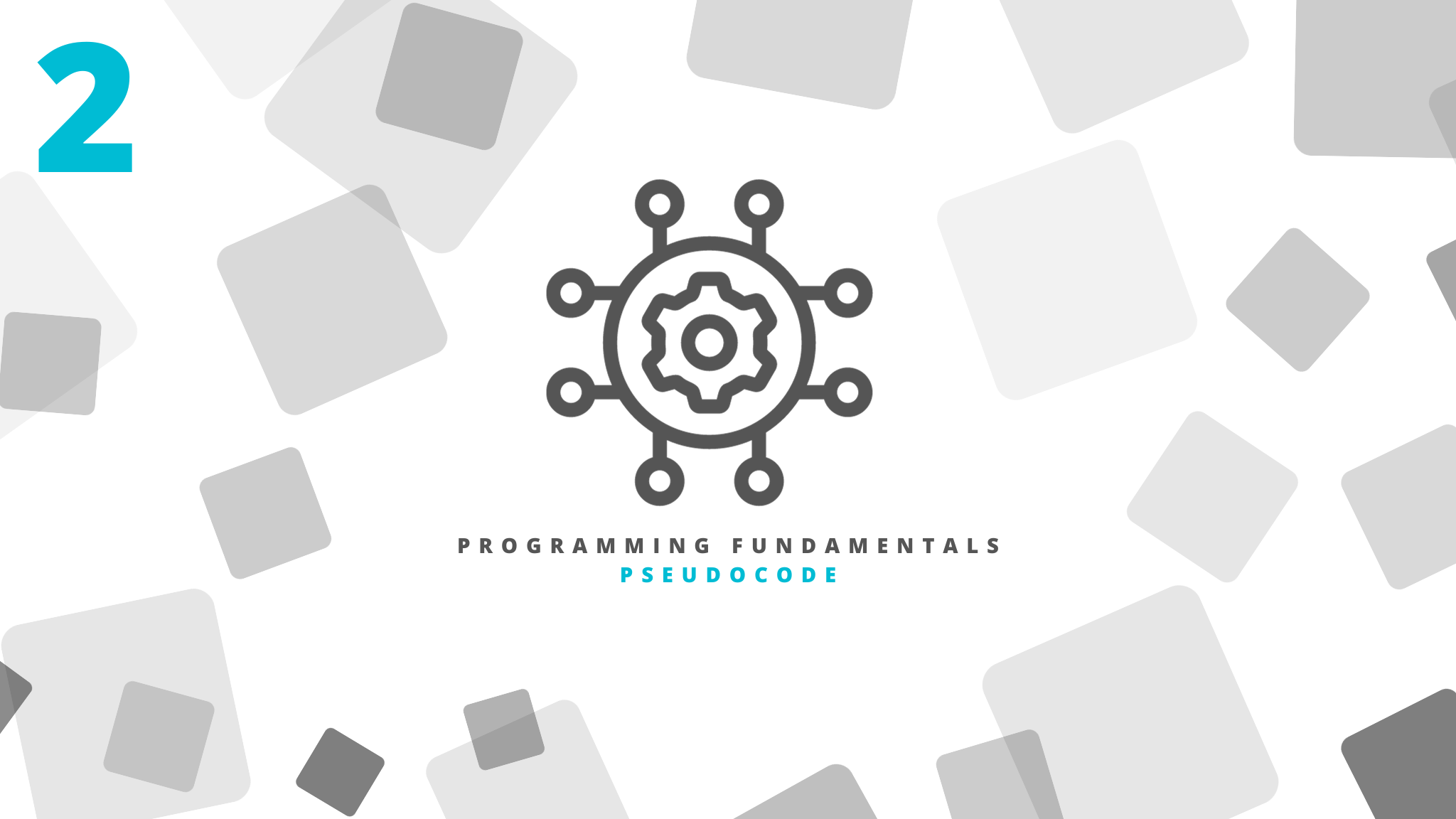
Programming Fundamentals Posts
- Algorithms
- Pseudocode (current)
In the last post we talked about how algorithms help us to define a set of instructions to solve a problem. Most of the course will be explained using algorithms so for ease we will represent algorithms using pseudocode.
Pseudocode
Pseudocode is a simplified specification language that is used as an intermediate tool between natural language and a specific programming language. It provides a structured and understandable way to express algorithms without the need to follow the strict syntax of a real programming language.
Pseudocode is used to describe the logic and steps of an algorithm clearly and concisely before implementing it in a programming language. For each example we will show a pseudocode and a implementation across many programming languages. This way, we can have a "generic" way to resolve a problem, and you can go and implement it later in any language of your preference.
Pseudocode Rules and Conventions
There are many standards of Pseudocode, I will use a generic one based on the syntax of Pascal. The purpose of pseudocode is not running it anyway, just understanding the algorithm that is behind. Here are some conventions we will use:
- Indentation: Indentation is used to indicate the structure and hierarchy of the code. Code blocks are indented with spaces or tabs to show their relationship.
- Comments: Comments are used to add notes and explanations to the code. They are denoted with the "#" symbol or "//".
- Assignment of Values: To assign a value to a variable, an arrow symbol, such as "<-" or "←", is used. For example, "x ←5" assigns the value 5 to the variable x.
- Arithmetic Operations: Pseudocode allows for basic arithmetic operations such as addition (+), subtraction (-), multiplication (*), and division (/). Parentheses can also be used to group operations.
- Declaration: Nothing can be used without declaring it, and variables must have a data type.
- Delimitation: Each algorithm is delimited with Algorithm and EndAlgorithm.
- End of Instruction: Instructions end with a semicolon (;).
- Identifiers: Identifiers must start with a letter and be written without spaces.
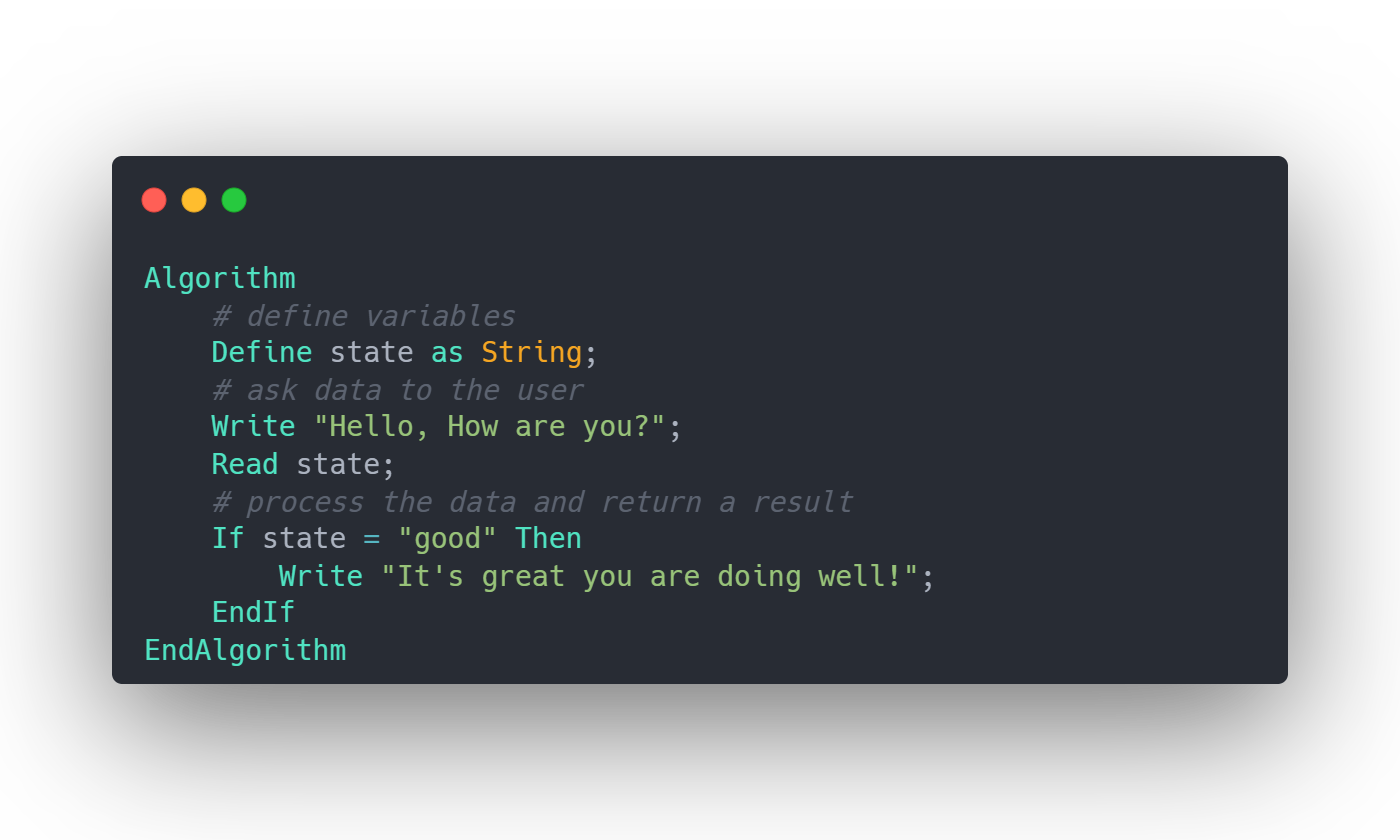
As I told you before, this is a generic language, so probably you will encounter other pseudocodes with other keywords and syntax. Don't worry, again, the idea of pseudocode is to have a language to communicate our algorithms clearly.
Programs
A program is a set of instructions written in a specific programming language that tells a computer how to perform a task or solve a problem. These instructions are written following a specific syntax and structure.
Parts of a program
A program typically consists of several parts which elements we will study throughout this course.
Input operations
They are usually located at the start of the program and indicate the dependencies and data requirements of the program.
- Declarations and imports: At the beginning of a program, declarations and imports can be included to allow the program to use external libraries or modules necessary for its operation.
- Data input: Programs often require interaction with the user or reading data from a file to obtain the necessary information for processing.
Processing
These are the elements that execute the operations over the input data to solve the problem and generating a result.
- Functions and procedures: A program can contain functions and procedures, which are blocks of code that perform a specific task. These functions and procedures can be called from other parts of the program to reuse code and improve organization.
- Variables and data structures: Programs use variables to store and manipulate data. These variables can be of different types, such as numbers, text strings, or more complex data structures like lists or dictionaries.
- Control statements: Control statements allow the program to make decisions and execute different actions based on certain conditions. These statements include conditional structures (such as if-else or switch) and loops (such as for or while).
Output operations
- Result output: After processing, the result is saved to a file, sent, displayed to the user, etc. to provide a solution to the problem at hand.
Types of instructions
The last theorical concept you have to know before going to the next steps is the different types of instructions. The types of instructions can vary according to the programming language, some of those are:
- Start and end: All instructions in a program are contained between the start and end. The start indicates the starting point of the program, while the end marks the end of execution. These start and end points may vary depending on the programming language used. This way, languages like Python the start and end of a block is delimited by the indentation, meanwhile C-based languages use braces.
- Branching: Branching instructions allow the program to take different paths of execution based on certain conditions. Some common examples of branching instructions are conditionals and loops that we will see in next posts.
- Assignment: Assignment instructions are used to assign a value to a variable.
- Input and output: Input and output instructions allow interaction with the user or reading/writing data from and to different sources.
Conclusion
Pseudocode is a tool to represent algorithms in a way that can be translated to any programming language with no complexes. We set some conventions and rules for the use of pseudocode, but it is just one way of the infinite standards of pseudocode there is out there.
Once we start writing our algorithm in a programming language, the set of instructions serve to create a program. Programs are usually divided in a start where user is requested to input data, a processing stage where computer executes operations whose result will we shown in the output stage.
Finally, we reviewed the different kind of instructions, serving this as introduction to the next post where we will see how the computer uses and represents data.
If you enjoy the content, please don't hesitate to subscribe and leave a comment! I would love to connect with you and hear your thoughts on the topics I cover. Your feedback is greatly appreciated!