Experimenting with the IMG Processing SDK
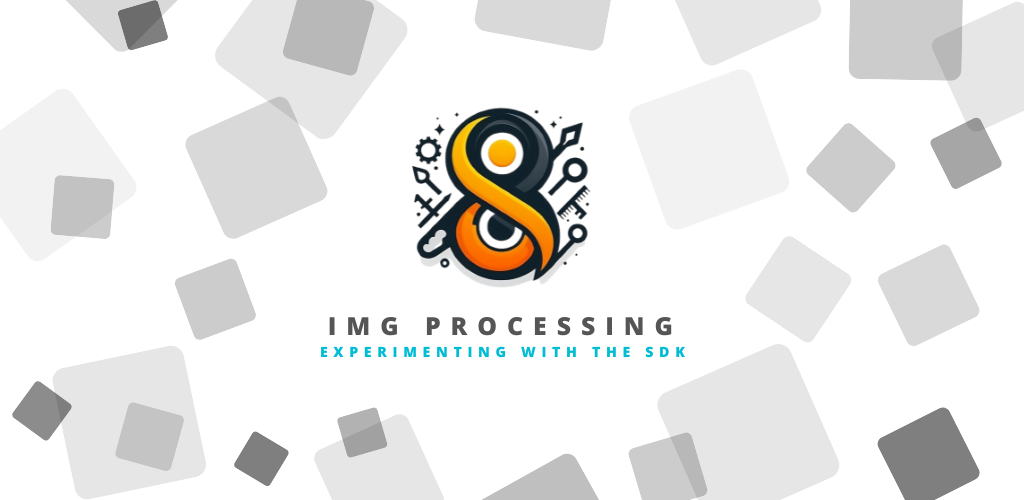
In the last article, we saw how to getting started with the IMG Processing API. It is a nice abstract alternative to interact with the IMG Processing, a powerful and flexible API and node SDK that provides a wide range of image manipulation and analysis capabilities.
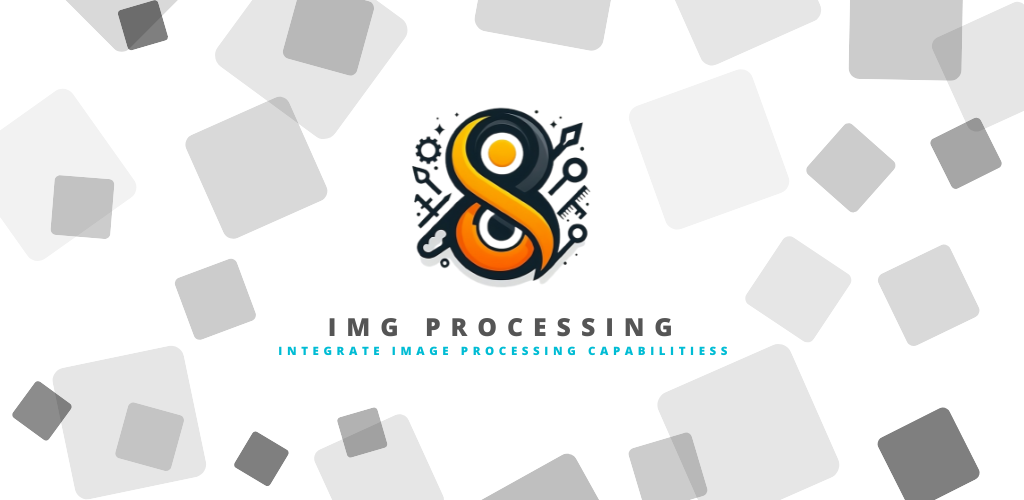
Today, I would like to share with you how to use the node-sdk.
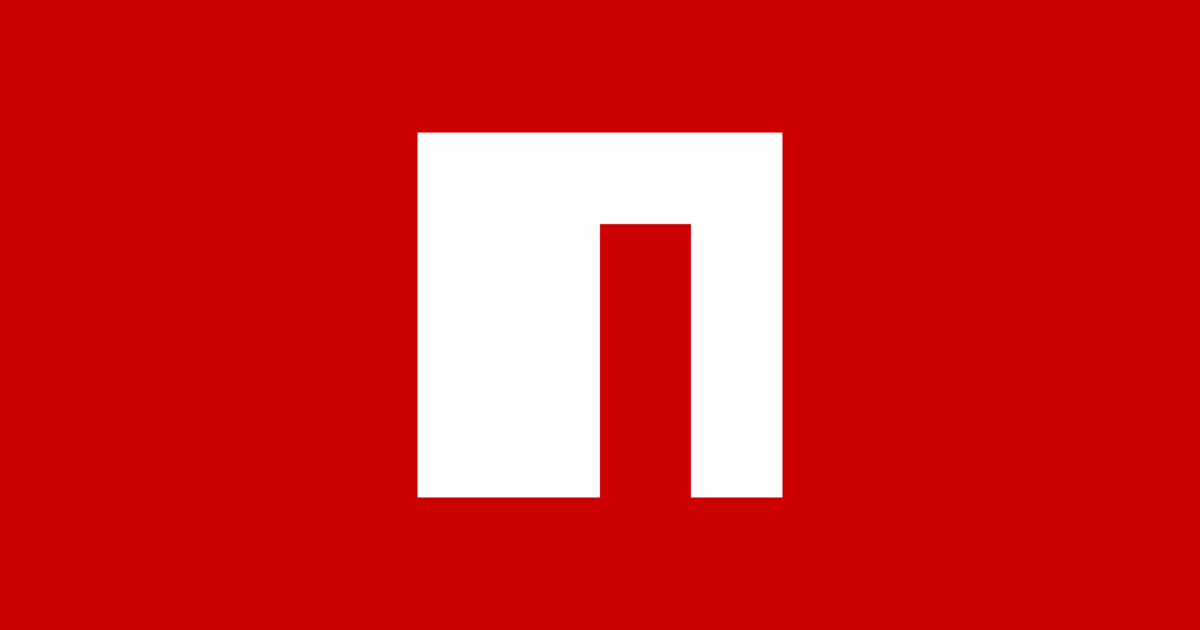
Getting Started
A Software Development Kit (SDK) makes easier the integration of the library in NodeJS based projects, this way with the API key, and a intuitive API, you are able to interact with IMG Processing without internal complications like handling HTTP requests and responses, parsing, etc.
Let's stop talking and see it in action. First install the SDK.
npm install img-processing-sdk
This library give us 3 main classes, the IMGProcessingClient
, ImageObject
, and IMGProcessingAPIError
. First, let's initialize the client with our API key:
import { IMGProcessingClient } from 'img-processing-sdk';
const client = new IMGProcessingClient({
apiKey: process.env.IMG_PROCESSING_API_KEY,
});
This give us an IMGProcessingClient
object, the main class of the IMG Processing SDK. It is a wrapper around http requests to the IMG Processing API, providing a simple and easy-to-use way to interact with the API. You can check the available methods in the documentation:

Exploring the SDK
Once you initialized the client, you can start interacting with the API using the same methods of the specification, for example, let's upload an image in our file system:
const image = await client.uploadImage({
image: 'path/to/image.jpg',
name: 'image.jpg',
});
Once we uploaded the image, the method returns us a ImageObject
. It represents an image processed using the IMG Processing API. The object contains information about the image, such as its URL, size, and format, like the following:
{
"id": "image_b3wfdnk9gjx442a6deayfh17",
"name": "image.jpg",
"url": null,
"width": 700,
"height": 320,
"format": "jpeg",
"size": 27696,
"created_at": "2024-09-22T16:00:29.700Z"
}
Additionally to those read only properties, the object contains many methods that you can review in the documentation:

For example, let's convert the image to PNG:
const pngImage = await image.convert({
format: "png",
});
Now we got the converted png image:
{
"id": "image_jwlx4c3p2djgrdrwrja23i74",
"name": "image.jpg",
"url": null,
"width": 700,
"height": 320,
"format": "png",
"size": 62504,
"created_at": "2024-09-22T16:04:14.078Z"
}
That's it, we converted the image from JPEG to PNG with no complexes. If you want to store the result, download the image and save it in the filesystem:
const blob = await pngImage.download();
The Next Steps
IMG Processing is still on a release candidate stage, so there could be some random errors that I'm still trying to find through an intensive automated testing.
If you find any error, you can create an issue in the GitHub repository:
During the next posts I will tell you more about of these changes, and keep you updated about the achievements and problems I encounter during this new journey. In the meantime, keep experimenting and creating amazing things!
If you enjoy the content, please don't hesitate to subscribe and leave a comment! I would love to connect with you and hear your thoughts on the topics I cover. Your feedback is greatly appreciated!